Qt Widgets
← Google Play Store | ● | Qt Signals And Slots →
The graphical user interface design of Qt is based on a widget hierarchy.
A widget is a C++ class object which represents a graphical element of the GUI. Each widget can be structured to contain child widgets organized in a specific layout. Thereby, a hierarchy of graphical elements is constructed by parent child relationships.
Each widget is derived from the base class “QWidget”. Its ctor takes a single argument, a pointer to the parent widget in the hierarchy. If a widget is destroyed its children are deleted automatically.
Different types of widgets are created by specializations of the base class QWidget. Qt includes specialized widgets like QPushButton, QComboBox etc. Here is a small subset of the entire Qt class hierarchy:
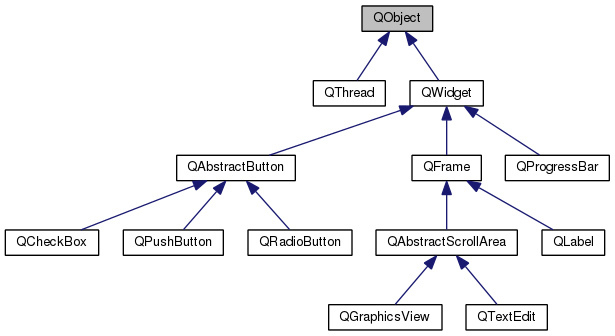
Simple example to create a specialized widget with three radio buttons:
class ThreeButtonWidget: public QWidget { public: ThreeButtonWidget(QWidget *parent = NULL) : QWidget(parent) { QBoxLayout *layout = new QBoxLayout; layout->setDirection(QBoxLayout::TopToBottom); layout->addWidget(new QRadioButton()); layout->addWidget(new QRadioButton()); layout->addWidget(new QRadioButton()); setLayout(layout); } ~ThreeButtonWidget() {} };
Main program that instantiates a respective widget:
#include <QApplication> #include "ThreeButtonWidget.h" int main(int argc, char *argv[]) { QApplication app(argc, argv); ThreeButtonWidget w; w.show(); return(app.exec()); }
← Google Play Store | ● | Qt Signals And Slots →