Qt-UI
Threaded Image Conversion
← Job Queue | ● | KDE4 Integration →
The application “DropPNG” accepts a drop event and starts an image conversion job for each dropped file:
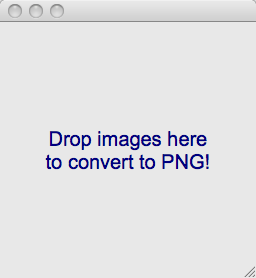
The image conversion jobs are executed in a background thread:
worker *thread = new worker;
When a drop event happens, the job queue of the background thread gets another job:
void dropEvent(QDropEvent *event)
{
const QMimeData *mimeData = event->mimeData();
if (mimeData->hasUrls())
{
event->acceptProposedAction();
QList<QUrl> urlList = mimeData->urls();
for (int i=0; i<urlList.size(); i++)
{
QUrl qurl = urlList.at(i);
QString url = qurl.toString();
if (url.startsWith("file://"))
{
url = url.remove("file://");
std::cout << "start job for " << url.toStdString() << std::endl; // debug
MyConverterJob *job = new MyConverterJob(url.toStdString());
thread->run_job(job);
}
}
}
}
{
const QMimeData *mimeData = event->mimeData();
if (mimeData->hasUrls())
{
event->acceptProposedAction();
QList<QUrl> urlList = mimeData->urls();
for (int i=0; i<urlList.size(); i++)
{
QUrl qurl = urlList.at(i);
QString url = qurl.toString();
if (url.startsWith("file://"))
{
url = url.remove("file://");
std::cout << "start job for " << url.toStdString() << std::endl; // debug
MyConverterJob *job = new MyConverterJob(url.toStdString());
thread->run_job(job);
}
}
}
}
When executing a job in the background thread, the file is loaded and saved as PNG:
class MyConverterJob: public Job
{
public:
MyConverterJob() : Job() {}
MyConverterJob(const std::string &s) : Job(s) {}
virtual ~MyConverterJob() {}
virtual int execute(worker *worker)
{
// get file name to be converted
QString filename(c_str());
// try to load file as image
QImage image;
if (!image.load(filename)) return(1);
// remove suffix from file name to yield output name
QString output = filename;
if (output.endsWith(".gif", Qt::CaseInsensitive)) output.truncate(output.size()-4);
if (output.endsWith(".jpg", Qt::CaseInsensitive)) output.truncate(output.size()-4);
if (output.endsWith(".tif", Qt::CaseInsensitive)) output.truncate(output.size()-4);
output.append(".png");
// save image as png
if (!image.save(output, "PNG")) return(2);
return(0);
}
};
{
public:
MyConverterJob() : Job() {}
MyConverterJob(const std::string &s) : Job(s) {}
virtual ~MyConverterJob() {}
virtual int execute(worker *worker)
{
// get file name to be converted
QString filename(c_str());
// try to load file as image
QImage image;
if (!image.load(filename)) return(1);
// remove suffix from file name to yield output name
QString output = filename;
if (output.endsWith(".gif", Qt::CaseInsensitive)) output.truncate(output.size()-4);
if (output.endsWith(".jpg", Qt::CaseInsensitive)) output.truncate(output.size()-4);
if (output.endsWith(".tif", Qt::CaseInsensitive)) output.truncate(output.size()-4);
output.append(".png");
// save image as png
if (!image.save(output, "PNG")) return(2);
return(0);
}
};
Access the full example via WebSVN:
Checkout the Qt example via SVN:
svn co svn://schorsch.efi.fh-nuernberg.de/qt-examples/example-07
← Job Queue | ● | KDE4 Integration →