VisExercises
Exercise 7
← Exercise 6 | ● | Exercise 8 →
Dicom Volume
Exercise (7a)
- Check out the dicom data directory from svn:
svn co svn://schorsch.efi.fh-nuernberg.de/dicom-data data
- Use the dicom/dicombase.h/.cpp module of the Qt frame work to load a series of DICOM images from the data directory via:
unsigned char *readDICOMvolume(const char *filename,
long long *width,long long *height,long long *depth,
unsigned int *components);
long long *width,long long *height,long long *depth,
unsigned int *components);
- Make sure that you link with DCMTK!
- Load example DICOM data from “data/MR-Kiwi/0*” or “data/MR-Artichoke/0*” or “data/CT-Cardiac/*.dcm”.
- The data is organized linearly in memory, which means that the cell array index for the left voxel of the first row is 0, the cell array index for the next voxel of the first row is 1, the cell array index for the left voxel of the next row is width, the cell array index for the next voxel is width+1, and so forth.
- For components==1, the data is organized linearly in memory with consequent bytes for each voxel.
- For components==2, the data is organized linearly in memory with consequent 16-bit LSB words for each voxel. Each 16-bit LSB word consists of a sequence of an 8-bit lo-byte and an 8-bit hi-byte. Therefore, the 16-bit value at the cell index c is data[2*c]+256*data[2*c+1].
Alternative Exercise (7b)
- Check out the data directory from svn:
svn co svn://schorsch.efi.fh-nuernberg.de/dicom-data data
- Use the pvm/ddsbase.h/.cpp module of the Qt frame work to load a PVM volume file from the data directory:
unsigned char *readPVMvolume(const char *filename,
unsigned int *width,
unsigned int *height,
unsigned int *depth,
unsigned int *components);
unsigned int *width,
unsigned int *height,
unsigned int *depth,
unsigned int *components);
Exercise (7c)
- Come up with a formula for the cell index based on index position (i, j and k).
- Print the center value of the dicom volume at index position (width/2, height/2, depth/2).
- Print the center value in percent of the maximum contained value.
- Check: The center value of the Kiwi data set at the index position (128,128,52) is about 30% of the maximum value. For the Artichoke data set the result is about 7% and for the CT cardiac data set we get about 5%.
Exercise (7d)
- Quantize 16-bit data to 8-bit data using the following function of the pvm/ddsbase.h/.cpp module:
unsigned char *quantize(unsigned char *volume,
long long width,long long height,long long depth,
bool msb);
long long width,long long height,long long depth,
bool msb);
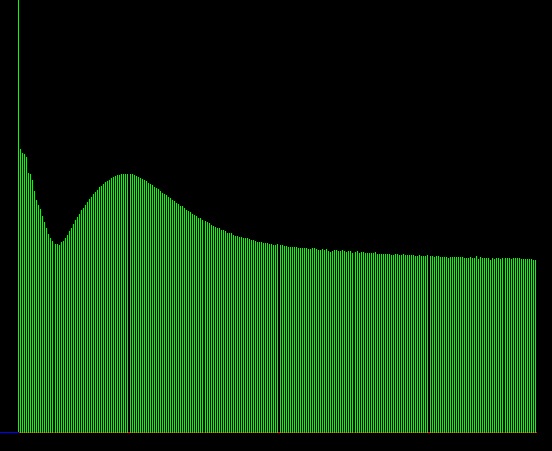
Exercise (7e)
- Count the voxels with zero value.
- Print the voxel count.
- Print the percentage of voxels with zero values compared to the voxel count of the entire volume.
Home work (7f)
- Count the number of voxels for each scalar value in a histogram table (that is 0..255 for 8-bit unsigned data).
- Plot the corresponding histogram curve with OpenGL.
- Make sure that it scales properly for different data sets.
The next exercise builds on this one.
← Exercise 6 | ● | Exercise 8 →